A simple Admin page to manage user list in Remix & MYSQL
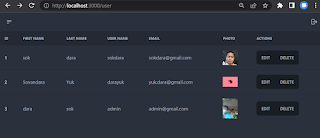
In the earlier tutorial, you learn to do authentication in Remix & MYSQL database . A visitor is able to create a new user account with profile photo and login to the remix app. Now we continue the tutorial by creating a simple admin page to manage user list. An authorized user will be able to see the user list, update an existing user, and remove the user from the database. In the user folder, create index.jsx file. The index.js file is the admin page. It lists all registered users and has buttons to edit and delete a specific user. import { json } from "@remix-run/node" ; import { useLoaderData } from "@remix-run/react" ; import { redirect } from "@remix-run/node" ; import {db} from "../../utils/db" ; import {getUserId} from "../../utils/session" ; export const loader = async ({request}) => { const uid = await getUserId(request); if ( ! uid) return redirect( 'user/login' ); try { let user